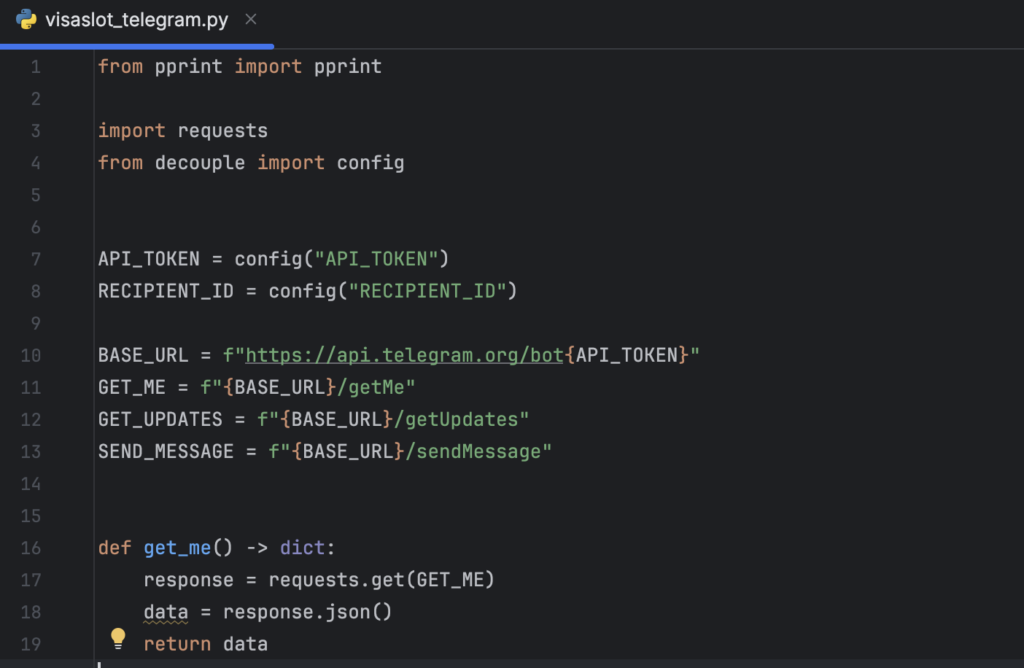
It’s my first time to hear of environment variables and wondered what they are or why they matter.
This guide will break down the basics of environment variables in a simple and easy-to-understand way.
What Are Environment Variables?
Environment variables are like helpers that make sure programs run smoothly without needing to hardcode settings directly into the program. This makes applications more flexible and easier to manage. Here’s how they help:
Set paths for programs: They tell your computer where to find certain programs.
Store configuration settings: They keep important details like database connections or API keys.
Control behaviour: They adjust how an application runs in different environments, like development, testing, or production.
Using a .env File and the Python Decouple Library
Managing environment variables can be a breeze with a .env
file and the python-decouple
library. This approach is straightforward and keeps your configuration clean and organised.
What is a .env File?
A .env
file is a simple text file that contains environment variables. Each line in the file is a key-value pair that looks like this:
DATABASE_URL=postgres://user:password@localhost:5432/mydatabase
SECRET_KEY=mysecretkey
DEBUG=True
Why Use the Python Decouple Library?
python-decouple
is a handy library that allows you to easily read these environment variables from a .env
file. It helps keep your settings separated from your code, making your application more secure and easier to manage.
How to Set It Up
- Install Python Decouple: First, you need to install the
python-decouple
library. You can do this using pip:
pip install python-decouple
- Create a .env File: In the root directory of your project, create a
.env
file and add your environment variables:
DATABASE_URL=postgres://user:password@localhost:5432/mydatabase
SECRET_KEY=mysecretkey
DEBUG=True
- Use Decouple in Your Python Code: Now, you can use
python-decouple
to read these variables in your Python code. Here’s an example:
from decouple import config
# Reading environment variables
DATABASE_URL = config('DATABASE_URL')
SECRET_KEY = config('SECRET_KEY')
DEBUG = config('DEBUG', default=False, cast=bool)
print("Database URL:", DATABASE_URL)
print("Secret Key:", SECRET_KEY)
print("Debug Mode:", DEBUG)
In this example:
config('DATABASE_URL')
reads theDATABASE_URL
from the.env
file.config('SECRET_KEY')
reads theSECRET_KEY
.config('DEBUG', default=False, cast=bool)
reads theDEBUG
variable and casts it to a boolean.
- Run Your Application: When you run your application,
python-decouple
will load the variables from the.env
file. This way, you can keep sensitive information out of your source code and easily change configurations by editing the.env
file.
Benefits
- Security: Sensitive data like passwords and API keys are kept out of your codebase.
- Flexibility: Change settings by simply editing the
.env
file. - Portability: Easily move your application between different environments (development, testing, production) by using different
.env
files.
Using a .env
file along with the python-decouple
library is a simple and effective way to manage your application’s configuration. It keeps your settings organised, secure, and easy to manage.
Conclusion
At first, they might seem confusing, but they really help manage my software. Whether I need to set paths, store secrets, or control how an app runs, environment variables work behind the scenes to keep things running smoothly.