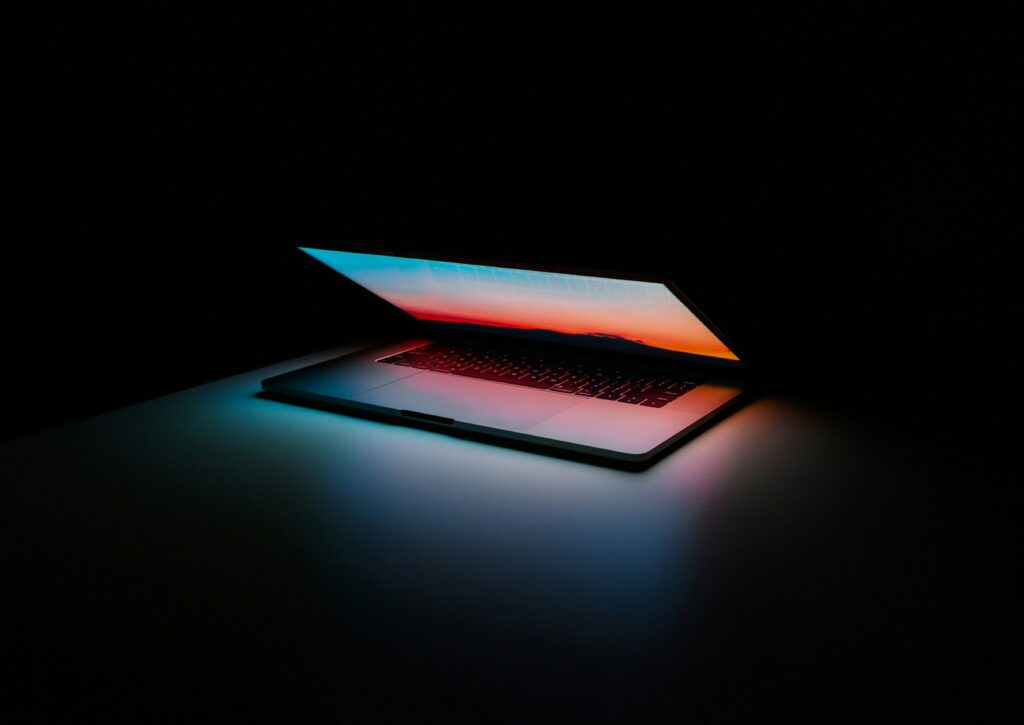
I learned C# in a module of my master’s degree in Mobile Apps and Wearables. Some aspects of C# feel similar to Python. Both C# and Python are supported by a range of powerful IDEs and editors, such as Visual Studio, Visual Studio Code, and PyCharm.
Personally, I use Visual Studio Code for C#, but for Python, I use PyCharm because some experts recommended it.
1. Core Programming Concepts
Variables and Data Types
In both C# and Python, you use basic data types like integers, floats, strings, and booleans. C# needs you to specify the type of each variable, while Python figures it out on its own.
- C#:
int x = 10;
double y = 3.14;
string name = "Jern";
bool isActive = true;
- Python:
x = 10
y = 3.14
name = "Jern"
is_active = True
Control Flow
Control flow in both languages is managed through similar constructs. You use if
, else if
/elif
, and else
for decision-making, and for
and while
loops for iteration.
Conditionals:
- C#:
if (x > 10) {
Console.WriteLine("x is greater than 10");
} else if (x == 10) {
Console.WriteLine("x is 10");
} else {
Console.WriteLine("x is less than 10");
}
- Python:
if x > 10:
print("x is greater than 10")
elif x == 10:
print("x is 10")
else:
print("x is less than 10")
Loops:
- C#:
for (int i = 0; i < 5; i++) {
Console.WriteLine(i);
}
int count = 0;
while (count < 5) {
Console.WriteLine(count);
count++;
}
- Python:
for i in range(5):
print(i)
count = 0
while count < 5:
print(count)
count += 1
2. Functions and Methods
Both C# and Python support functions for organising code into reusable blocks.
- C#:
public int Add(int a, int b) {
return a + b;
}
int result = Add(3, 4);
Console.WriteLine(result);
- Python:
def add(a, b):
return a + b
result = add(3, 4)
print(result)
3. Object-Oriented Programming
Both languages support OOP principles, such as creating classes, defining methods, and managing object properties.
- C#:
public class Person {
public string Name { get; set; }
public int Age { get; set; }
public void Greet() {
Console.WriteLine($"Hello, my name is {Name} and I am {Age} years old.");
}
}
Person p = new Person { Name = "Jern", Age = 31 };
p.Greet();
- Python:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
p = Person("Jern", 31)
p.greet()
4. Exception Handling
Error handling with try-catch
in C# and try-except
in Python follows similar principles.
- C#:
try {
// code that may throw an exception
}
catch (Exception ex) {
Console.WriteLine($"Error: {ex.Message}");
}
- Python:
try:
# code that may throw an exception
except Exception as ex:
print(f"Error: {ex}")
5. Data Structures
Both languages offer comparable data structures for storing and manipulating collections.
Lists/Arrays:
- C#:
List<int> numbers = new List<int> { 1, 2, 3, 4 };
- Python:
numbers = [1, 2, 3, 4]
Dictionaries/Maps:
- C#:
Dictionary<string, int> ages = new Dictionary<string, int> {
{ "Soju", 30 },
{ "Judy", 25 }
};
- Python:
ages = {"Soju": 30, "Judy": 25}
6. Libraries and Frameworks
Using libraries is a common practice in both languages, though the syntax for including them differs.
- C#: Uses
using
directives.
using System.Collections.Generic;
- Python: Uses
import
statements.
import math
7. File I/O
Reading from and writing to files is straightforward in both languages.
- C#:
using System.IO;
File.WriteAllText("example.txt", "Hello, World!");
string content = File.ReadAllText("example.txt");
- Python:
with open("example.txt", "w") as file:
file.write("Hello, World!")
with open("example.txt", "r") as file:
content = file.read()
8. Asynchronous Programming
Handling asynchronous tasks with async
and await
is supported in both languages.
- C#:
async Task<string> FetchDataAsync() {
await Task.Delay(1000); // Simulate async work
return "Data";
}
- Python:
import asyncio
async def fetch_data():
await asyncio.sleep(1) # Simulate async work
return "Data"